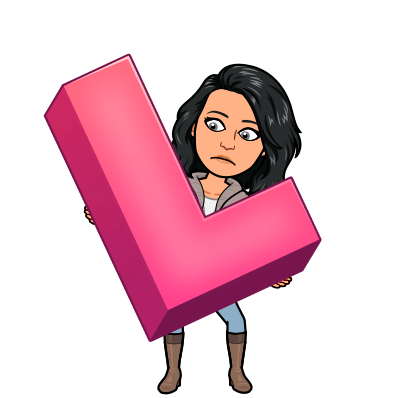
You’ve been given an array of words and asked to sort them in alphabetical order.
const words = ['red', 'yellow', 'green']
words.sort()
console.log(words) // 'green', 'red', 'yellow']
Wow, that was easy!
Here’s how it works:
The sort() method is used to rearrange the elements of an array in ascending order by default. However, the default sorting behavior is based on converting elements to strings and comparing their UTF-16 code unit values. In UTF-16, each character is represented by a code unit, which is a 16-bit binary number.
Here are the UTF-16 code unit values for the lowercase English alphabet characters:
‘g’ – 103
‘y’ – 121
‘r’ – 114
These values represent the order of characters as per their ASCII values. When using the sort()
method, it compares the UTF-16 code unit values, which is why ‘green’ comes before ‘red’ and ‘yellow’ in the sorted result.
Beware when sorting lowercase and uppercase words or letters.
const colors = ['red', 'Yellow', 'green']
colors.sort()
console.log(colors) // result: ["Yellow", "green", "red"]
Why didn’t this work?
Sorting is case-sensitive, and uppercase letters will be sorted before lowercase letters. That’s because uppercase letters have lower Unicode code points than lowercase letters.
Here are the UTF-16 code unit values for the uppercase English alphabet characters:
‘g’ – 103
‘Y’ – 89
‘r’ – 114
That’s why 'Yellow'
comes before 'green'
and 'red'
in the sorted result. So if you directly use sort()
on an array of strings, it might not always give you the expected result!
To avoid this from happening, use a custom function instead!
const colors = ['red', 'Yellow', 'green']
colors.sort(function(a,b) {
return a.toLowerCase() < b.toLowerCase() ? -1 : 1
})
console.log(colors) // result: [green, red, Yellow]
The comparison function takes two parameters, a and b, which represent two elements being compared.
a.toLowerCase()
andb.toLowerCase()
convert the strings a and b to lowercase, ensuring a case-insensitive comparison.
The comparison is based on the result of comparing the lowercase versions of a and b. Here’s how it works:
- If a (in lowercase) is less than b (in lowercase), the ternary conditional returns
-1
, indicating that a should come before b in the sorted array. - Otherwise, if a (in lowercase) is greater than or equal to b (in lowercase), the ternary conditional returns
1
, indicating that a should come after b in the sorted array.
Let’s refactor!
const colors = ['red', 'Yellow', 'green']
colors.sort((a,b) => a.toLowerCase() < b.toLowerCase() ? -1 : 1)
console.log(colors) results: ['green', 'red', 'Yellow']
We can refactor again using the localeCompare() method!
The localeCompare()
method in JavaScript is a built-in string method used to compare two strings based on their linguistic and natural language rules. It provides a way to perform string comparisons that take into account various language-specific considerations, including case sensitivity!
Here’s the refactored code using localeCompare():
const colors = ['red', 'Yellow', 'green']
const compare = (a, b) => a.toLowerCase().localeCompare(b.toLowerCase())
colors.sort(compare)
console.log(colors) results: ['green', 'red', 'Yellow']
Remember: the sort() method modifies
the original array!