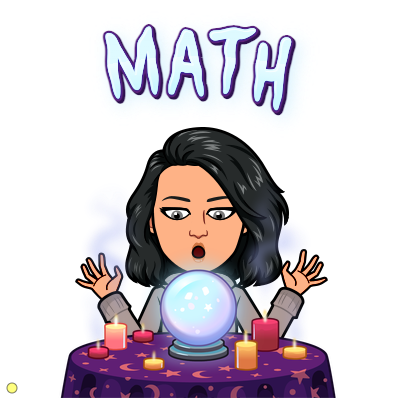
You’ve been given an array of numbers and asked to sort them in ascending order. Let’s take a look…
const numbers = [9, 10, 4]
numbers.sort()
console.log(numbers) // result: [10, 4, 9]
Well, that’s not right!
Here’s why:
The default behavior of the sort() method in JavaScript is to convert elements to strings before performing comparisons. This can sometimes lead to unexpected results when sorting numbers.
Instead, you’ll need to use a comparison function that explicitly compares numbers like this:
const numbers = [9, 10, 4]
numbers.sort((a, b) => a - b)
console.log(numbers) // [4, 9, 10]
How does the compare function work?
It takes two arguments (usually named a and b), representing two elements being compared. It should return a negative value if a should be sorted before b, a positive value if a should be sorted after b, and zero if the order of a and b doesn’t matter.
Let’s explain:
Sort 1: [9, 10, 4], where a = 9 and b = 10. Since 9 – 10 = -1, a should be sorted before b: 9 is sorted before 10, there’s no change. [9, 10, 4]
Sort 2: [9, 10, 4], where a = 10 and b = 4. Since 10 – 4 = 6, a should be sorted after b: 10 is sorted after 4. [9, 4, 10]
Sort 3: [9, 4, 10], where a = 9 and b = 4. Since 9 – 4 = 5, a should be sorted after b: 9 should be sorted after 4. [4, 9, 10]
FYI: the sort() method modifies
the original array!
To avoid this from happening, use the slice() method to create a shallow copy:
Below, the slice() method is called on originalArray with no arguments. This creates a new array that is a shallow copy of originalArray. The purpose of using slice() in this context is to prevent the sort() method from directly modifying the originalArray. Since sort() sorts arrays in place and modifies the original array, creating a shallow copy using slice() ensures that the original array remains unchanged while you work with the sorted version!
const originalArray = [9, 10, 4]
const sortedArray = originalArray.slice().sort((a,b) => a-b)
console.log(originalArray) // result: [9, 10, 4]
console.log(sortedArray) // result: [4, 9, 10]